Displaying Theme Data with WordPress
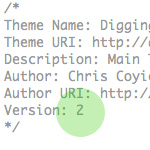
A cool trick you can do with WordPress is display information directly from your theme’s style.css
stylesheet. I recently used this on a site where the theme’s version number is used throughout the template to keep things current and consistent.
get_theme_data()
The function that makes it possible is called get_theme_data(), and it simply returns an array of information about any of your theme files. Here is how it’s used in your theme template:
get_theme_data()
is deprecated as of WP 3.4. For this technique, use wp_get_theme() instead.<?php get_theme_data( $theme_filename ); ?>
So $theme_filename
is required and should be the path and filename of your theme’s style.css
file. There is no default value for this, so you need to ensure a proper value.
So what information can you display with get_theme_data()
? The function returns an array of values that basically comprises the different meta items in your stylesheet. Here is a list of possible return values (all strings):
Name
– theme nameTitle
– either theme name or linked theme nameURI
– theme URIDescription
– wptexturized version of the theme nameAuthorURI
– theme author URITemplate
– theme parent template, if existsVersion
– theme version numberStatus
– theme status (default:publish
)Tags
– theme tagsAuthor
– author name or linked author name
Note that these return values are case-sensitive and will not work if the first letter is not capitalized.
Examples
The WordPress Codex provides the following example for getting and displaying the theme Name
and Author
:
<?php
/*
* Assign theme folder name that you want to get information.
* make sure theme exist in wp-content/themes/ folder.
*/
$theme_name = 'twentyeleven';
/*
* Do not use get_stylesheet_uri() as $theme_filename,
* it will result in PHP fopen error if allow_url_fopen is set to Off in php.ini,
* which is what most shared hosting does. You can use get_stylesheet_directory()
* or get_template_directory() though, because they return local paths.
*/
$theme_data = get_theme_data( get_theme_root() . '/' . $theme_name . '/style.css' );
echo $theme_data['Title'];
echo $theme_data['Author'];
?>
This should get you going.. just copy/paste into your theme template and indicate the theme name in that first line of code. Displaying other bits of theme data is matter of editing/replicating those last two lines.
Once you see how it works, you can do cool stuff with it, like display your theme’s version number sort of globally throughout your site. I’m using this technique to append version parameters to my stylesheet URLs, like so:
<link rel="stylesheet" href="<?php bloginfo('stylesheet_directory'); ?>/style.css<?php if(function_exists('theme_version')) theme_version(); ?>">
..and the output markup looks something like this (notice the appended version parameter, ?v=1.3
):
<link rel="stylesheet" href="http://example.com/wp-content/themes/xycss/style.css?v=1.3">
Because I am using multiple stylesheets, this method really saves a LOT of time trying to keep track of everything. Here is the theme_version()
function that goes in your theme’s functions.php
file:
// display version number
function theme_version() {
$theme_name = 'xycss'; // customize with your theme name
$theme_data = get_theme_data( get_theme_root() . '/' . $theme_name . '/style.css' );
echo '?v=' . $theme_data['Version'];
}
And with a few modifications, you can also display your theme’s information in your posts and pages via shortcode:
// version number shortcode
function theme_version_shortcode() {
$theme_name = 'xycss'; // customize with your theme name
$theme_data = get_theme_data( get_theme_root() . '/' . $theme_name . '/style.css' );
return $theme_data['Version'];
}
add_shortcode('theme_version', 'theme_version_shortcode');
With that second snippet in your functions.php
file, displaying your theme info directly in pages is as easy as writing this:
[theme_version]
..and the output:
1.3
It’s pretty cool stuff, and I’m sure there’s tons more you can do with it too. If you know any tricks or tips, please share in the comments, Thanks :)
13 responses
-
That’s brilliant. How does one find this? by accident? :P
-
hehe, thinking to build the first WP theme, maybe until the year’s gone. Good snippet to print the ownership! Now I just have to build it!
-
I was just trying to do this. I was thinking of using this in the copyright section and including the theme name, author name, and author’s URI. It would be great for a framework.
-
Nice topic. It’s a good idea to store these for easy re-use down the road in the theme, here’s a modified snippet of what I’ve been using…
// grab the theme data array $my_theme_data = get_theme_data(TEMPLATEPATH . '/style.css'); define('MY_THEME_NAME', $my_theme_data['Title']); define('MY_THEME_AUTHOR', $my_theme_data['Author']); define('MY_THEME_URI', $my_theme_data['URI']); define('MY_THEME_VERSION', $my_theme_data['Version']); define('MY_THEME_INFOLINE', MY_THEME_NAME . ' by ' . MY_THEME_AUTHOR . ' (' . MY_THEME_URI . ') v' . MY_THEME_VERSION); // usage in register style wp_register_style('my_custom_style', get_bloginfo( 'stylesheet_url' ),false, MY_THEME_VERSION, 'screen');
-
Quite handy. I’ll for sure need to bookmark this for further down the road.
-
Couldn’t you use get_current_theme() get the current theme name?
-
On second thoughts, how about using get_template_directory() or get_stylesheet_directory() to get the server directory of the template
-
If your code is in functions.php file then you can simply do this:
$theme_data = get_theme_data( dirname(__FILE__) . '/style.css' );
and you can also improve your shortcode by:
/* theme data shortcode Usage: [theme_data] OR [theme_data code=Author] etc */ function theme_data_shortcode($atts, $content=null) { extract( shortcode_atts( array('code' => 'Version' ), $atts ) ); $theme_data = get_theme_data( dirname(__FILE__) . '/style.css' ); return $theme_data[$code]; } add_shortcode('theme_data', 'theme_data_shortcode');
-
Hi,
I can’t find in my Theme “get_theme_data” in functions. I am using a theme called Hatch. Can you help me???
PLEASE!!!
-
-
hi guys, how i can rename this premium template?
this dont has nothing in funcitions only sending for include.php who i send here. i try to rename the folder and syle.css but dont work i got a frase, dont rename this :(
if someone could helpme, i really need this..
$theme_data = get_theme_data(TEMPLATEPATH .'/style.css'); $theme_cache = wp_upload_dir(); define('THEMENAME', $theme_data['Title']); define('THEMEPREFIX', strtolower(substr(THEMENAME, 0, 2))); define('THEMEAUTHOR', $theme_data['Author']); define('THEMEVERSION', $theme_data['Version']); define('THEMECACHE', $theme_cache['basedir'] . '/cache'); define('THEMOLUTION', TEMPLATEPATH . '/themolution'); define('THEMEINCLUDES', TEMPLATEPATH . '/themolution/includes'); define('THEMELANGUAGES', TEMPLATEPATH . '/themolution/languages'); define('THEMEJS', TEMPLATEPATH . '/themolution/assets/js'); define('THEMECSS', TEMPLATEPATH . '/themolution/assets/css'); define('THEMEIMAGES', TEMPLATEPATH . '/themolution/assets/images'); define('SCJS', TEMPLATEPATH . '/themolution/shortcodes/js'); define('SCCSS', TEMPLATEPATH . '/themolution/shortcodes/css'); define('SCIMAGES', TEMPLATEPATH . '/themolution/shortcodes/images');
-
This looks like a good trick to have on hand. Bookmarking, will be sure to tell others about it! Thanks for the tip!
-
FYI,
get_theme_data
has been deprecated since v.3.4.Use wp_get_theme instead.