Including jQuery in WordPress (The Right Way)
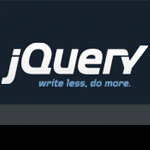
If you want, you can just download jQuery, put it on your server and link to it from your header.php
file in the <head>
section. But that can cause you grief. For one thing, some plugins use the jQuery library, and they are going to load it as well. This can cause problems. How was your plugin to know you already had it loaded?
Update!
Much has changed with WP script loading since this article was posted. These days, WordPress automatically loads jQuery for you, whenever some other script requires it. The smart loading requires that you specify jquery
as the $deps
parameter when enqueuing your other JavaScript files, for example:
wp_enqueue_script('my-custom-script', get_template_directory_uri() .'/js/my-custom-script.js', array('jquery'), null, true);
This declares jQuery as a dependency for my-custom-script
, so WordPress automatically will load its own copy of jQuery. For reference, here are the parameters used for wp_enqueue_script():
wp_enqueue_script( $handle, $src, $deps, $ver, $in_footer )
So when we declare our custom script, we set the $deps
parameter equal to array('jquery')
and call it a day.
Now, let’s say that we want to use a version of jQuery that is different than the one that is included with WordPress. We could simply enqueue it, but then there would be two copies/versions of jQuery loaded on the page (yours and WP’s). So before we enqueue our own version of jQuery, we must de-register the WP version. Here is the final code to make it happen:
// include custom jQuery
function shapeSpace_include_custom_jquery() {
wp_deregister_script('jquery');
wp_enqueue_script('jquery', 'https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js', array(), null, true);
}
add_action('wp_enqueue_scripts', 'shapeSpace_include_custom_jquery');
Here we use wp_deregister_script() to deregister WP’s jQuery before including our own version, which for this example is the Google-hosted jQuery library, version 3.1.1
. You will of course want to change the $src
parameter to match the URL of whatever jQuery script you want to use.
So that’s the current “right way to include jQuery in WordPress”. One important note for theme developers: publicly released themes should use WP’s jQuery and not de-register it. Just makes life easier in the long run.
Original post
… continued from intro …
Another thing is that WordPress already includes a copy of jQuery. Here is how you can load up jQuery in your theme the smart (and intended) way. Put the following code in your header.php
file in the <head>
section:
<?php wp_enqueue_script("jquery"); ?>
<?php wp_head(); ?>
Your theme probably already has the wp_head
function, so just make sure you call the wp_enqueue_script
function BEFORE that. Now you are all set to call your own jQuery JavaScript file, AFTER the wp_head
function.
<script type="text/javascript"
src="<?php bloginfo("template_url"); ?>/js/yourScript.js"></script>
You are ready to rock, but there are still some considerations. For example, safeguarding yourself from the future possibility of conflict with other libraries. You really shouldn’t be using multiple JS libraries to begin with, but… better safe than sorry.
To be super-safe, you can put jQuery into “no conflict” mode and use a different shortcut for jQuery. In this case “$j” instead of the default “$”. The standard “$”, for example, can conflict with Prototype. Here is an example of a safe bit of jQuery JavaScript:
var $j = jQuery.noConflict();
$j(function(){
$j("#sidebar li a").hover(function(){
$j(this).stop().animate({
paddingLeft: "20px&"
}, 400);
}, function() {
$j(this).stop().animate({
paddingLeft: 0
}, 400);
});
});
Can you recognize this bit of code? We use it right here at DigWP.com1 to do the cool “link nudging” in the sidebar!
1 Editor’s note: the link nudge demo has been removed (it was part of an older theme design).
48 responses
-
Seems there is a small problem in the code.
The
"
should be"
.Still, useful post, thanks!
-
What about using jQuery hosted by Google?
-
Yes, this is one of the reasons I hate to use to insert stuff.
-
Thare’s a plugin that replaces those codes and load the files from Google. I think it’s called Use Google Libraries.
Combine that with this method and you have both a clean and fast way to load jquery and other js. :)
-
-
-
Jeff, I believe that WP already puts its version of jQuery into no-conflict mode by default, as there is a legacy of using Prototype for WP in the past to think of.
Otherwise, congrats on the launch of the site, and good luck with the self-publishing!
-
Does the WordPress copy of jQuery reflect the current version available on jQuery’s site?
-
As of this writing, WordPress includes jQuery 1.3.2. Whenever jQuery comes out with a new stable version, WordPress adds it to the next update. More or less.
-
-
For some reason this method doesn’t work for me. If I include jQuery with the script tag everything work as expected. This thing is driving me crazy :(
-
for some reason – mine won’t work with the wp_enqueue_script – but with the regular it does. any ideas?
-
-
Good post, we need plugin developers to stop including their own so you end up having 6 instances of jquery being loaded on your WP sites…
-
I think the problem is usually on themes more than plugins :/
If only it was possible to make WordPress itself check for any manual calls to jquery and other frameworks and replace them with wp_enqueue_script()..
-
-
Isn’t it also a good idea to use the version google spreads, or the latest version via jquery instead of the own hosted jquery-instance. That way, if more sites use this method, the js-file gets cached and makes the page load faster ..
grtz,
Tom.-
There’s a plugin for WordPress called “Use Google Libraries”. If you want to use that, do so. It’ll replace the references used by WordPress with the Google hosted versions.
Using that plugin and doing the enqueue approach is the best way, because the enqueue approach takes care of dependencies, loads things in the correct order, and ensures that plugins and themes don’t conflict with each other by only loading each library once.
-
-
Good idea, but it’s probably still better IMHO to load a minified version of jQuery and not the unpacked full source.
I personally pull it from Google Libraries and use a remove_action() for those plugins that load it again. In all reality thought, I don’t use a lot of plugins that use jQuery other than the ones Envato commissions third parties for, or the ones I write myself. So I guess my situation is not cookie cutter.
In any case, I can see this being useful though in situations where you might not have as much control as you like over jQuery being loaded in your theme.
-
WordPress uses the minified version of jQuery automatically, unless you have it set to “developer” mode, in which case it loads the full version.
As for using Google libraries, there’s a plugin for that: “Use Google Libraries”.
-
-
Nice to know, I hadn’t done this because I stick all my JS just before the closing body tag. If plugin authors were to insert their JS into the wp_footer tag area, we’d be onto a real winner performance-wise (YSlow).
Thanks and good luck with this project!
-
Great article, I am going to start using these instead of uploading the same jQuery to each of my domains, thanks guys! Great site!
-
What about the various jquery plugins. Is there a proper way to load them in WordPress?
-
What does template_url means? Plese can you give an example..
-
That’s a function that wordpress uses to print your theme directory.
That means that
/js/yourScript.js
translates tohttp://example.com/wp-content/themes/theme/js/yourScript.js
More info about bloginfo() parameters.
-
-
oh wow – super useful post! i’ve been dreading doing this cos i thought it would take ages, but you’ve just made it so easy. :) thanks heaps!
-
Great article and helped me solve numerous problems and conflicts. I was having an instance when I could not close a modal dialog in FF but in IE it worked fine.
-
Followup: You can also add other dependencies. If one dependency is already loaded (by a plugin, for example), wordpress will not load it twice.
wp_enqueue_script(‘handle’, ‘path/to/js’, array(‘jquery’, ‘prototype’), ‘version’, false);
will load your script “handle”, jQuery, and Prototype, all in the head section.
-
What’s the boolean argument at the end? I only see four arguments in the wp_enqueue_script documentation in the Codex.
-
-
WordPress’ wp_enqueue_script function can also load your own script for you that depends on jQuery, without making two calls to wp_enqueue_script. There is also a bool at the end that tells WordPress to load your script with the wp_head hook or the wp_footer hook. (In the example, the true as the last argument in wp_enqueue_scripts tells the script to load in the footer section. If you set this to false, it will load in the headers section.)
-
You can also use the closure pattern to sandbox your code like this :
(function($){
$(function(){
//your onload code
});
})(jQuery);
It’s totally safe and avoids breaking some plugins which rely only on the $ variable.
Anyway, another good reason to use enqueue is it’s much more easier to unqueue them remotely from a custom plugin/theme. I personnally removed them with hooks to unload some KB of display.
Also, it’s even better to make those scripts shrinked and minimized for faster responses.
:-)
-
Just what I needed. Thanks for this one!
-
Has anybody had any problems with this? I have replaced my call to jquery with the method in this post and for some unknown reason it isn’t working.
I’ve put the wp_enqueue call before the wp_head and my script.js after.
When I look at the source code all of the scripts are in the right place but still no good. If I go back to my own copy of jquery it works again. Hmmm!
I really want to use this method, its a lot cleaner and I love doing things the ‘right’ way.
Anybody else ran into problems?
-
This is great, gonna make it easier for me to do wordpress themes with jquery stuff, without making the user mess with getting jquery. I’m bad at writing instructions, so anything that saves me from that, heh.
Awesome tip.
-
I have tried this trick every which way ! and it doesn’t work for me using WP 2.8.2. My theme just works like jQuery wasn’t loaded and checking with FF it looks like it is loaded ! Any ideas why ? and how to make it work so I don’t load jQuery twice.
Thanks
-
I have tried this trick every which way ! and it doesn’t work for me using WP 2.8.2 and your code exactly as advised above.
My theme just works like jQuery wasn’t loaded and checking with FF it looks like it is loaded !
Any ideas why ? and how to make it work so I don’t load jQuery twice.(which works but slows loading pages )
Thanks-
jean: If your jQuery code uses the
$shortcode
, wrap it in something like this:jQuery(document).ready(function($){
// your $ code here
});
Then it’ll work.
-
-
Thank you for the tips. They’ve gotten me part of the way there. But, like jean I have tried this every which way and just cannot get it to work. I am using it in a plugin and I understand from another blog that these techniques apply.
The line in the plugin that was causing me problems is as follows:
echo "\n";
I commented it out and instead wrote this solution solved the conflict problem, but now the plugin doesn’t work. Any help you could give me would be much appreciated. thanks.
-
I lied! the conflicts are still there and the plug in doesn’t work. Help! I’ve literally spent days trying to solve this.
-
-
I am having a hard time loading jquery-ui-core and jquery-ui-tabs. Had no problems loading jquery, using:
jQuery(document).ready(function($){
// your $ based code here will work fine
});
to load the jflow plugin, but can’t get simple tabs to work in my theme. Anyone else have problems with wp_enqueue and jquery-ui-core?
-
hmmm. Well, I got it to work – love a fresh start on a new day. I am using the following:
WP 2.7 – and using wp_enqueue to load jquery, jquery-ui-core, jquery-ui-tabs, and jflow. I had to load jquery first, since jflow depends on it. Loaded jflow Second, then loaded the ui-core and ui-tabs in that order. I swear i tried this a million times last night to no avail, but it seems happy now…
I thought maybe the ordering was causing the problem – perhaps it was just a cache issue. Good luck all! Great blog by the way…
-
-
I have also noticed that you can run a condtional statment on the function that imports
-
if (wp_enqueue_script('jquery')) echo 'Works';
else echo 'Failed';
-
Trying to get it all to work with woothemes premium news. Seems like a bit of a nightmare! Have tried so many combos of whats suggested.
Installing some plugins that call jquery break the theme.
-
I should mention I’m not very skilled with jquery or javascript :P. Can it be dumbed down any more for those like me? Like there are multiple references to jquery throughout the theme and some plugins, am I meant to replace them?
-
-
I’m a bit confused about the no-conflict stuff. I know what the no-conflict function does, and I see what the enqueue WP function does. But what I don’t understand is what the end result of WP being in “no-conflict mode” by default is?
If I set my no-conflict string to be $foo, then every time I want to use a jQuery function I should use $foo(function()). Yet before reading this article I had been using WP’s included jQuery (loaded with enqueue) and just $(function()).
I guess the question is really this:
If WP puts jQuery into no-conflict mode, what is the custom string I’m supposed to use?
-
I am just experimenting with a new design, since the current one is a bit old (been using it the past 3 years). So far I hesitated to implement new themes and functions since I am really no expert when it comes to css, etc. I struggled quite some time to get jquery activated, but the adive in this post and in the post helped me out quite a bit!
Thanks a lot!
-
wonderful and helpful information
thank you so much !!!