How to Include Styles in WordPress Child Themes
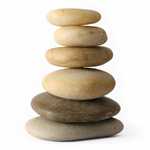
This DigWP tutorial explains the “new” way to include parent stylesheets in Child Themes. I put the word “new” in quotes because the technique actually has been around for years, but there are many developers and designers who still use the old @import
way of adding parent styles. This tutorial is for people who may be unfamiliar with using WordPress’ enqueue functionality for Child Themes.
Here you’ll find copy-n-paste techniques, examples, caveats, and numerous resources. Basically everything you need to know about including styles in your Child Themes. Let’s dig in..
The old way
If you’ve been around WordPress (or web development) for awhile, you’re probably familiar with the following technique for including a parent theme’s stylesheet:
/*
Theme Name: Example Child Theme
Template: themedirectory
*/
/* import default parent styles */
@import url("../themedirectory/style.css");
/* add child theme styles below */
So you just use the @import
query in the Child Theme’s style.css
and done. That way the parent stylesheet is included, and you can customize the Child Theme as desired by adding your own styles.
This method still works in general, but there are some gotchas and caveats now with certain browsers and JavaScript frameworks. Plus the performance aspect of using @import
is not optimal.
The new way
As performance issues and other downsides of using @import
were discovered, developers began using the enqueue method to include parent styles in Child Themes. You probably already are familiar with enqueueing scripts and styles:
function twentysixteen_scripts() {
// enqueue style
wp_enqueue_style('twentysixteen-style', get_stylesheet_uri());
// enqueue script
wp_enqueue_script('twentysixteen-script', get_template_directory_uri() .'/js/functions.js', array('jquery'), '20150825', true);
}
add_action('wp_enqueue_scripts', 'twentysixteen_scripts');
This example is taken from the current default WordPress theme, Twenty Sixteen. It shows how the wp_enqueue_scripts
hook is used for enqueueing both scripts and styles. This is the recommended way of adding scripts and styles to any WordPress theme, including Child Themes.
Enqueue parent styles in child themes
Just as we use the enqueue method for parent themes, we also should use it for child themes. So instead of using @import
in your child theme’s style.css
file, you can add the following code to your child theme’s functions.php file:
// enqueue styles for child theme
// @ https://digwp.com/2016/01/include-styles-child-theme/
function example_enqueue_styles() {
// enqueue parent styles
wp_enqueue_style('parent-theme', get_template_directory_uri() .'/style.css');
}
add_action('wp_enqueue_scripts', 'example_enqueue_styles');
This function includes the parent theme’s stylesheet in the Child Theme. There are several parameters that may be defined when using wp_enqueue_style()
; check out the resources at the end of this tutorial for more information.
So again, instead of using the @import
query to include the parent stylesheet, use the enqueue method instead. Both techniques are simple enough, but enqueueing is optimal in most cases due to performance concerns, compatibility issues, and other nuanced gotchas.
Download the Example Child Theme
To see this technique for enqueueing parent styles in action, check out my related post at WP Mix, WordPress Example Child Theme. It’s a free download that works on any WordPress-powered site. Provides a dead-simple way to see how to create your own Child Theme.
Bonus: Enqueue parent and child stylesheets
To flesh this tutorial out a bit more, here is a function that will add both the parent and child stylesheets to the Child Theme. You can add this function to your child theme’s functions.php file:
// enqueue styles for child theme
// @ https://digwp.com/2016/01/include-styles-child-theme/
function example_enqueue_styles() {
// enqueue parent styles
wp_enqueue_style('parent-theme', get_template_directory_uri() .'/style.css');
// enqueue child styles
wp_enqueue_style('child-theme', get_stylesheet_directory_uri() .'/style.css', array('parent-theme'));
}
add_action('wp_enqueue_scripts', 'example_enqueue_styles');
When added to your Child Theme, this function will include both parent and child stylesheets. Notice that we’re defining the dependency parameter in the child-theme enqueue, array('parent-theme')
. This tells WordPress that the child styles are dependent on the parent styles, so WordPress will load the parent styles first. This is just a precaution, as the order in which the enqueue functions are called should dictate the correct order.
To enqueue or not to enqueue
Should you enqueue child styles as well as parent styles? Is it necessary? This is where a lot of confusion exists regarding enqueueing stylesheets in child themes. The answer depends on how the parent styles are included in the parent theme. For example, if the parent theme is using get_stylesheet_uri()
to enqueue styles, for example as in Twenty Sixteen:
wp_enqueue_style('twentysixteen-style', get_stylesheet_uri());
..then you don’t need to enqueue the child styles. This is because get_stylesheet_uri()
returns the URL of the current active theme, which in this case is the child theme.
Best advice if there is any confusion is to add something like this in the parent theme’s functions template:
// conditional enqueue styles
function example_enqueue_styles() {
$deps = false;
if (is_child_theme()) {
$deps = array('parent-styles');
// load parent styles if active child theme
wp_enqueue_style('parent-styles', trailingslashit(get_template_directory_uri()) .'style.css', false);
}
// load active theme stylesheet
wp_enqueue_style('theme-styles', get_stylesheet_uri(), $deps);
}
add_action('wp_enqueue_scripts', 'example_enqueue_styles');
This function checks if the current theme is a child theme. If so, it loads the parent styles and the child styles. If the current theme is not a child theme, only the parent styles are loaded. Notice the handling of the dependencies parameter, $deps
, which is set to false if the child theme is not active.
Register then enqueue
If you are developing themes for distribution, it is considered best practice to first register your stylesheets before enqueueing them. For example:
wp_register_style('child-theme', get_stylesheet_directory_uri() .'/style.css', array('parent-theme'));
wp_enqueue_style('child-theme');
Registering scripts and styles provides greater flexibility and can help to keep your codes nice and tidy.
Disabling a stylesheet
If you need to disable a parent style, you can do this:
// disable parent theme styles
function example_disable_parent_styles() {
wp_dequeue_style('parent-theme');
}
add_action('wp_enqueue_scripts', 'example_disable_parent_styles');
If you a different slug is used for the parent styles, you’ll need to change parent-theme
accordingly. See the resources at the end of this tutorial for more details.
URL reference
For your information, here are the URLs output by the various functions we’re using in this tutorial:
get_stylesheet_uri() = http://example.com/wp-content/themes/example-child/style.css
get_template_directory_uri() = http://example.com/wp-content/themes/example-parent
get_stylesheet_directory_uri() = http://example.com/wp-content/themes/example-child
I find this info can be super useful when diagnosing and testing different configurations, themes, and so forth.
Resources
Last but not least, here are the official Codex articles for the functions used throughout this tutorial:
4 responses
-
Great article but I think there needs to be a clarification. In the section about URL references, these two statements are incorrect:
get_template_directory_uri() = http://example.com/wp-content/themes/example-parent/style.css get_stylesheet_directory_uri() = http://example.com/wp-content/themes/example-child/style.css
Those two calls will NOT return style.css as part of the return value, only the path UP TO the style.css part will be returned (minus the trailing slash)
-
Super useful information, I have read many other articles regarding the same topic but something was always missing. You have done a good job BRO. Keep it up for beginners just like me. I am surely going to use the 2016 with a child theme now.
-
Great article jeff, really informative and on point.