How to Redirect Logged-In Users with WordPress
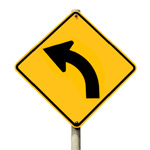
WordPress provides a variety of functions to redirect logged-in users. So which is best? It really depends on your goals; they’re all good methods, it just depends on what you’re trying to accomplish.
In this DigWP tutorial, we explain each of these methods along with some useful tips and tricks along the way. These techniques enable you to redirect logged-in users to internal pages, external pages, and even return them to the current page. They’re some great tools to have in the ’ol belt. So let’s dig in..
wp_redirect
The wp_redirect function is for redirecting all users to any absolute URI. Absolute URIs are basically full URLs and look like this:
https://example.com/book/
https://example.com/wp-content/images/redirecting-users.jpg
ftp://example.com/transfer/
To redirect users with wp_redirect
, place the following PHP snippet in your theme file:
<?php wp_redirect('http://example.com/'); exit; ?>
The parameters for wp_redirect
are two in number:
- $location = The absolute URI to which the user will be redirected. No default.
- $status = The status code to use. For example,
301
,302
, etc. The default is302
.
You can use template tags for the $location
parameter, for example:
<?php // redirect to the home page
wp_redirect(home_url()); exit; ?>
<?php // redirect back to current page
wp_redirect(get_permalink()); exit; ?>
To use a status code other than the default 302
, specify it like so:
<?php wp_redirect('http://example.com/', 301); exit; ?>
That code will send the user to example.com
along with status code 301
(Moved Permanently). The default 302
should be used for temporary redirects. Learn more about status codes.
auth_redirect
Next up, we have auth_redirect, which is a “simple function that requires that the user be logged in before they can access a page.” So for example, let’s say you have a “downloads” page that’s meant for logged-in users only. To prevent unauthorized access, we would add the following code to the top of the downloads page template:
<?php auth_redirect(); ?>
This extremely useful function checks whether or not the current user is logged in, and redirects them to the Login Page if not. By default, the user will be redirected back to the page from whence they came (e.g., the downloads page). Currently this function accepts no parameters, but having something like $location would make it even more awesome ;)
wp_logout_url
The wp_logout_url function returns the URL of your site’s Logout Page. But it can also redirect the user to any URL using the $redirect
parameter, like so:
<?php echo wp_logout_url($redirect); ?>
$redirect
parameter is a string containing the redirect URL. Here are some examples:
<!-- Logout & redirect to the home page -->
<a href="<?php echo wp_logout_url(home_url()); ?>">Logout</a>
<!-- Logout & redirect to current page -->
<a href="<?php echo wp_logout_url(get_permalink()); ?>">Logout</a>
<!-- Logout & redirect to specific URL -->
<a href="<?php echo wp_logout_url('http://example.com/'); ?>">Logout</a>
The automatic logout link is great, but the subsequent redirect is a super-useful tool that we use in our custom login/register tutorial.
is_user_logged_in
Lastly we have the is_user_logged_in function, which technically doesn’t do any redirecting, but enables you to check if the user is logged in. Here is a simple example that should work in any theme template file:
<?php if (is_user_logged_in()) { echo "logged in"; } else { echo "not logged in"; } ?>
The is_user_logged_in
function returns TRUE
or FALSE
, depending on the user’s status. So to display custom content to logged in users, you could do something like this:
<?php if (is_user_logged_in()) { ?>
<p>Welcome, registered user!</p>
<?php } else { // not logged in ?>
<p>Welcome, visitor!</p>
<?php } ?>
And you can mix-n-match is_user_logged_in
with other template tags and PHP snippets to return more refined results. For example, we can serve custom content based on logged status and location:
<?php if (is_user_logged_in()) {
if (is_page()) {
echo "Logged-in user visiting a page";
} elseif (is_feed()) {
echo "Logged-in user viewing a feed";
} elseif (is_search()) {
echo "Logged-in user doing a search";
} else {
echo "Logged-in user doing something else";
}
} else { // user is not logged-in
if (is_page()) {
echo "Normal visitor on a page";
} elseif (is_feed()) {
echo "Normal visitor viewing a feed";
} elseif (is_search()) {
echo "Normal visitor doing a search";
} else {
echo "Normal visitor doing something else";
}
} ?>
This example demonstrates the flexibility of WordPress conditional tags and how is_user_logged_in
throws another layer of control to the mix.
Note about auth_redirect & is_user_logged_in
As discussed here, auth_redirect
currently seems to be acting kinda weird, where the post-login redirect isn’t working. Instead of returning the user to the current page after login, the user always ends up back at the Login Page. It looks like the auth_redirect
function isn’t recognizing the new “logged-in” user status, and so it just keeps sending the user back to login.php
.
So until this is fixed, there is a simple workaround which involves combining auth_redirect
with is_user_logged_in
to do what auth_redirect should be doing. All that’s required is the following snippet of code placed at the top of your theme template:
<?php if (!is_user_logged_in()) { auth_redirect(); } ?>
With this technique, auth_redirect
executes only if the user is NOT logged in. So yeah it’s a hack, but it works great and should continue working if/when auth_redirect
is fixed.
9 responses
-
Thanks, very concise and timely!! I was just trying to use wp_redirect for the first time and ran into some weirdness in terms of where I hooked into it. I ended up having to call it in wp_footer so that visitors going to the www version of the site got redirected to the correct page. You can see my WordPress StackExchange post here – I’d love to learn if anyone understands why this was happening or a better way to write/call the function.
Thanks again!
-
wp_redirect() has to be called before the page has loaded. I had this same problem recently and after a lot of searching discovered why. I also updated the Codex page.
-
-
Thanks for this Jeff. All the admin and user management stuff is super helpful. If you could post anything about customizing the registration email, password recovery email, and how to also customize your own profile that would be awesome (maybe redirecting non admin users from the dashboard too :).
I know there’s plugin for this stuff (theme my login, theme my profile, and dashboard redirect) but it would be great to see the code and figure out how to do it on my own. Thanks again!
-
Hello Man Just Going To AsK U About Your Voting Pluging That u Yousing
Whats Here Name I Need It Very Much
Thanks Man
-
Extremely helpful article. auth_redirect(); will definitely come in handy one of these days.
-
Hi Jeff,
Thanks for the post, it’s useful as always!
I am having a problem with one of my clients’ sites, which needs to have some pages accessible only by members. However the parent page of the protected area is still visible to non-members!
I am using the Member Access plugin (it works on all other pages, except for the parent page), but even when trying to add the code:
<?php if (!is_user_logged_in()) { auth_redirect(); } ?>
or<?php auth_redirect(); ?>
..it doesn’t redirect the users to the login page, if they click on the button for accessing the protected area of the site.
This is the website. And the big blue “Tom Thumb Parent Login” button is where you should only be able to access if you’re logged in.
Do you have any idea why this is happening?
Thanks a lot.
Cintia
-
You should probably mention that wp_redirect() needs to be called before the page loads or it won’t work. I went round and round with this issue a couple of weeks ago because it wasn’t mentioned anywhere in the Codex. Needless to say, after I figured that out it was smooth sailing. I also updated the Codex with that information.