Customize Your WordPress Dashboard
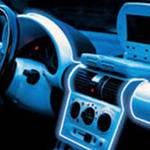
There are many ways to customize the WordPress Dashboard. Over the years, the Dashboard has evolved into a highly flexible information portal, enabling an overall, big-picture view of the main components of your site, while also providing granular data on everything from recent comments and plugin updates to incoming links and WordPress news. And that’s just the default functionality, there are also a ton of dashboard widgets and plugins available in the WordPress Plugin Directory that you can use to transform your Dashboard into just about anything, or even disable it completely.
The easiest way to customize your Dashboard
For many WordPress sites, you can achieve complete Dashboard nirvana by simply selecting a few options in the Dashboard’s Screen Options panel. On the Dashboard, click the tab in the upper-right corner to toggle open your Dashboard configuration options. There you can choose your Dashboard preferences:
- Choose the widgets you want to show on the Dashboard
- Choose how many columns to use for the screen layout
Here is a screenshot illustrating this awesome functionality:
The ability to hide or show any default widgets makes it easy to set things up on the fly. Maybe your client doesn’t need the community news feed? Disable it. Maybe you’re getting some fatal errors (e.g., “Allowed memory size of 33554432 bytes exhausted”) for the Incoming Links and Plugins widgets?
You could spend time on the issue or you could just disable any widgets giving you grief. For my personal sites, I like to disable everything except for Right Now, Recent Comments, and Incoming Links, and display them in two columns. Keeps the Dashboard clean and fast.
But to each their own! That’s the beauty of WordPress :)
Customizing the Dashboard using plugins
Most of the time, the built-in Screen Options are all you need to customize your WordPress Dashboard. For more control over which users actually see the dashboard and its widgets, here are three great plugins (among many):
- My own creation, Dashboard Widgets Suite
- KP Design’s WP Hide Dashboard
- sant0sk1’s Clean WP Dashboard
Let’s take a closer look at each of these Dashboard plugins..
Dashboard Widgets Suite
Dashboard Widgets Suite streamlines your Admin Area by reducing the number of plugins required for widgets. It provides an entire set of awesome widgets that you can add to your WordPress Dashboard:
- Control Panel – Enable/disable widgets directly from the Dashboard
- User Notes – Add, edit, delete notes for any user role
- Feed Box – Display and customize any RSS Feed
- Social Box – Display social media links from Twitter, Facebook, etc.
- List Box – Display a custom list of links created via the Menu screen
- Widget Box – Display any theme or WP widget (e.g., Meta, Search, Text)
- System Info – Display system info (e.g., WP, PHP, server, client)
- Debug Log – Display, customize, reset your WP debug log
- PHP Error Log – Display, customize, reset your server error log
Each widget includes its own set of options for customizing display. The notes widget is super awesome, designed for serious note takers :)
WP Hide Dashboard
The WP Hide Dashboard plugin really cleans things up by removing the Dashboard and Tools menu, the Screen Options section, and even the Help button on the Profile page. It also prevents Dashboard access to users assigned to the Subscriber role. It’s also a breeze to use: just install and it works – no configuration necessary.
Clean WP Dashboard
The Clean WP Dashboard plugin doesn’t remove any of the Dashboard menus, Screen Options, or Help button, but it does enable you to remove any of the following Dashboard widgets for all users:
- Right Now
- Incoming Links
- Plugins
- Recent Comments
- QuickPress
- Recent Drafts
- WP Dev Blog
- Other WP News
With this plugin, disabling any of these default widgets also removes their corresponding display option from the Screen Options menu, but leaves all the settings and menus in place for other widgets. This also is a very simple plugin to use: just enable, pick your widgets, and done. Here is another screenshot to help illustrate how this plugin is useful for customizing your dashboard:
Even with WordPress’ default Screen Options and helpful plugins such as these, there are times when you’ll want to do even more with the customization of your Dashboard. Fortunately, with WordPress you’re not limited to default functionality and widgets – by adding a few lines of code to your theme’s functions.php file, you can make just about anything happen.
Customizing the Dashboard with functions.php
There are many cool ways to customize your WordPress site using your theme’s functions.php
file. The benefits of using functions.php
include:
- Direct control over site functionality
- Follows your theme to other sites (portability)
- You don’t need to rely on a plugin
- Set it and forget – no upgrades or fiddling
I would argue that in some cases, it’s actually easier to use functions.php
than it is to hunt down, install, configure, and manage a plugin. Just grab a code snippet (or write your own) and paste it anywhere into your theme’s functions.php
file. Test well and you’re done.
Let’s check out some functions.php
snippets useful for customizing the WordPress Dashboard.
Create your own Dashboard widget
You can display just about anything in your Dashboard. If default tools and other plugins aren’t getting you there, WordPress makes it easy to create your own. The easiest way to get started is to add the following to functions.php
:
// example custom dashboard widget
function custom_dashboard_widget() {
echo "<p>Dearest Client, Here’s how to do that thing I told you about yesterday...</p>";
}
function add_custom_dashboard_widget() {
wp_add_dashboard_widget('custom_dashboard_widget', 'How to Do Something in WordPress', 'custom_dashboard_widget');
}
add_action('wp_dashboard_setup', 'add_custom_dashboard_widget');
Here we are using two functions to do the job. The first one contains the content of the custom widget. The second one turns the output of the first function into a widget. And finally, we run the second function when the Dashboard is set up. Once this code is in place, visit your Dashboard. You should see your custom widget displayed like so:
Once you see how things work, it’s just a matter of adding the content and functionality. You could add a calendar, list recent subscribers, import feeds, display images, post tutorials, and just about anything else imaginable.
Disable default widgets
You can also use functions.php
to disable default Dashboard widgets without a plugin. Using the code found in the WordPress Codex, you would add this to your functions.php
file:
// disable default dashboard widgets
function remove_dashboard_widgets() {
global $wp_meta_boxes;
unset($wp_meta_boxes['dashboard']['normal']['core']['dashboard_right_now']);
unset($wp_meta_boxes['dashboard']['normal']['core']['dashboard_recent_comments']);
unset($wp_meta_boxes['dashboard']['normal']['core']['dashboard_incoming_links']);
unset($wp_meta_boxes['dashboard']['normal']['core']['dashboard_plugins']);
unset($wp_meta_boxes['dashboard']['side']['core']['dashboard_quick_press']);
unset($wp_meta_boxes['dashboard']['side']['core']['dashboard_recent_drafts']);
unset($wp_meta_boxes['dashboard']['side']['core']['dashboard_primary']);
unset($wp_meta_boxes['dashboard']['side']['core']['dashboard_secondary']);
}
add_action('wp_dashboard_setup', 'remove_dashboard_widgets');
This works fine, but it seems better to use the WordPress API. Specifically, we can use remove_meta_box() to clean up default dashboard widgets:
// disable default dashboard widgets
function disable_default_dashboard_widgets() {
remove_meta_box('dashboard_right_now', 'dashboard', 'normal'); // Right Now
remove_meta_box('dashboard_recent_comments', 'dashboard', 'normal'); // Recent Comments
remove_meta_box('dashboard_incoming_links', 'dashboard', 'normal'); // Incoming Links
remove_meta_box('dashboard_plugins', 'dashboard', 'normal'); // Plugins
remove_meta_box('dashboard_quick_press', 'dashboard', 'side'); // Quick Press
remove_meta_box('dashboard_recent_drafts', 'dashboard', 'side'); // Recent Drafts
remove_meta_box('dashboard_primary', 'dashboard', 'side'); // WordPress blog
remove_meta_box('dashboard_secondary', 'dashboard', 'side'); // Other WordPress News
// use 'dashboard-network' as second parameter to remove widgets from network dashboard
}
add_action('admin_menu', 'disable_default_dashboard_widgets');
I’m using this code to clean up the Dashboard of my eChunks site, and it works like a dream. You can also set the code to display the default widgets only for Admins by replacing the last line with this conditional snippet:
if (!current_user_can('manage_options')) {
add_action('wp_dashboard_setup', 'disable_default_dashboard_widgets');
}
This code basically says, “disable the default Dashboard widgets only if the user can’t manage options. So Admins will see the widgets but everyone else will not.
Disable plugin and other non-default widgets
If you recall from the second screenshot in this article, only the Simple:Press widget was displayed in the Dashboard. Even with the default widgets disabled, widgets from plugins and the functions.php
file are displayed. As with the default widgets, you can simply hide these widgets using the Screen Options panel, or you can completely disable them using a few lines code in your functions.php
file.
To disable a non-default Dashboard widget, you first need the name of the widget, which is available in the source code as one of the <div>
id
s. For example, the Simple:Press widget is wrapped in the following markup:
<div id="sf_announce" class="postbox">
And so the name of the widget is “sf_announce
”. Likewise, our custom Dashboard widget is wrapped with this:
<div id="custom_dashboard_widget" class="postbox">
Once you have the name of the widget you would like to remove, just add another line to the disable_default_dashboard_widgets()
function provided above, for example:
// disable default dashboard widgets
function disable_default_dashboard_widgets() {
// disable default dashboard widgets
remove_meta_box('dashboard_right_now', 'dashboard', 'normal'); // Right Now
remove_meta_box('dashboard_recent_comments', 'dashboard', 'normal'); // Recent Comments
remove_meta_box('dashboard_incoming_links', 'dashboard', 'normal'); // Incoming Links
remove_meta_box('dashboard_plugins', 'dashboard', 'normal'); // Plugins
remove_meta_box('dashboard_quick_press', 'dashboard', 'side'); // Quick Press
remove_meta_box('dashboard_recent_drafts', 'dashboard', 'side'); // Recent Drafts
remove_meta_box('dashboard_primary', 'dashboard', 'side'); // WordPress blog
remove_meta_box('dashboard_secondary', 'dashboard', 'side'); // Other WordPress News
// disable Simple:Press dashboard widget
remove_meta_box('sf_announce', 'dashboard', 'normal');
}
add_action('admin_menu', 'disable_default_dashboard_widgets');
Other Dashboard widgets may be disabled in the same way. Just replace sf_announce
with the name of your widget. If you have a lot of widgets to disable, you may not feel like digging through source code to decipher their names. Fortunately, there’s an easier way to get that information.
List all Dashboard widgets
To get a list of all active Dashboard widgets, add the following snippet to your theme’s functions.php
file:
// list active dashboard widgets
function list_active_dashboard_widgets() {
global $wp_meta_boxes;
foreach (array_keys($wp_meta_boxes['dashboard']['normal']['core']) as $name) {
echo '<div>' . $name . '</div>';
}
}
add_action('wp_dashboard_setup', 'list_active_dashboard_widgets');
Then go to your Admin and hit refresh. At the top of the page, you will see a list of all active widgets. Note that this method is a little rough because the list is basically spit out before all of the content, so make sure you’re not executing on a production site. Or be quick about it. Or use the conditional current_user_can
code from above. Either way, this is the easiest way to grab a list of all active Dashboard widgets.
Wrap up
What’s the take-home message from all of this? WordPress makes it easy to customize your Dashboard in three ways: default Screen Options, plugins, and functions.php
code. Using any combination of these three methods, you have complete control over the appearance and functionality of your WordPress Dashboard.
14 responses
-
Angry Birds Website?! Whoa!
-
Thanks for this tipps! At the moment I’m using the “Clean WP Dashboard” Plugin, but I think I’m going to try some other ways in my next project …
-
One thing I’ve been doing is creating a dashboard widget containing one of Facebook’s social plugins, so when I log into my blogs I can get a quick overview of how many times my posts have been shared.
-
This is just what I was looking for, thanks!
-
Nice stuff, not something ive EVER noticed before, lol… sigh. Dont have any imediate use to customise it, but will bookmark for alter ^_^
-
The dashboard has never been a dashboard. You would think it would allways be at the top like a dashboard.
As for altering it. Can you go more indepth. Like how woothemes changes theirs for example the tumblr esque themes totally alter the posting area and have that one area in the dash board to send post type as categories.
But hacking it to do post type would be better.
-
This is really a great post. I recently found out about this on my website. Thanks again.
-
Wow, thanks for this post. I found it really interesting, and more importantly useful. I have been using WordPress for a while now on my site. Quick Need and katskinner.com, and never actually realized you could customize your dashboard (at least not without some coding).
I like to use plugins on my dashboard such as analytics tracking (which saves me opening another website page or program if I am in a hurry to see any major changes). However with so many dashboard widgets piling up (and only thinking that my real option was to minimize windows I didn’t care for), this post has really helped me solve my dilemma.
Thank you, keep the great posts coming!
-
can i have my installed widgets on my dashboards ?
-
Useful tips, thanks!
Would it be possible to add a thumbnail of the featured images to the Posts Dashboard. And if so, could you show how to do that or point me in the right direction?
Thanks.
-
Hey, thanks Jeff, especially for the use of remove_meta_box to take out the dashboard widgets. I’ve been using the unset method which always seemed a little nasty.
Do you know of a way to set the default “Number of Columns” with a filter or function of some kind? I always end up setting it to 1 for CMS installs to keep it as clean as possible but whenever a new user is added, it sets it to two.
Thanks!
-
I must say I’ve been trying to find some good blog post covering this – there is less quality than you think on the Interent. Perhaps I shouldn’t be surprised :-). Anyway, happy to come across your article. Just what I was looking for. Just wanted to say thanks :)
-
I started messing around with this to add a feed for updates to my theme into the dashboard but I’m failing miserably:
function wpfolio_dashboard_widget() { echo "<p>Below is the latest news, click on a title to read the full entry.</p>"; $rss = @fetch_feed('http://wpfolio.visitsteve.com/wiki/?feed=rss2'); if ( isset($rss->items) && 0 != count($rss->items) ) { echo '<h3>WPFolio News</h3>'; } } function add_wpfolio_dashboard_widget() { wp_add_dashboard_widget('custom_dashboard_widget', 'WPFolio News', 'wpfolio_dashboard_widget'); } add_action('wp_dashboard_setup', 'add_wpfolio_dashboard_widget');
What am I missing here?